Tips for Android Developers to reduce APK file Size
1) ProGuard
A tool for code shrinking, like ProGuard, will significantly reduce APK file size. The tool is available at sourceforge. Note that, it is very important to re-test all of the application after applying ProGuard since it may change the application behavior sometimes. As ProGuard replaces the application symbols, to make the code difficult to read, it is important that you retain the symbol mapping, so that you can translate a stack trace back to the original symbols if you have to investigate a crash in your application.
2) Removal of Debug information
I recommend that you remove all debug-related functionality from the application. The application generally does not see or use this data, and the Android operating system does not require it to run the application. Hence, the debug information only wastes space, and should be removed.
To accomplish this, all debug related functionality must be enclosed in conditional blocks, like below:
static final debug = false; if (debug) { v(TAG, "Debug …”) }
It is important that the debug flag is set at compile time (i.e. declared as static final) for the compiler to be able to completely remove all debug functionality. Creating your own debug method like the one shown below is not a good idea because the call to myDebugPrint() is not enclosed by a conditional block, which means that the compiler must retain information about myDebugPrint() in the calling class.
public void myDebugPrint() if (Debug) v(TAG, "Debug …”); } } … myDebugPrint()
3) Removal of debug symbols from native libraries Using debug symbols makes sense if your application is still in development, and still requires debugging. But if debug symbols are still appearing when you compile a release build, and if you want to remove them, then we recommend that the Debug symbols be removed from native libraries (.so files). This is done using the arm-eabi-strip command, from the Android NDK.
4) Recommended media formats If your application relies heavily on images, audio or video, another way you can reduce APK file size is by using certain media formats. We recommend that you use the following media formats for images, audio and video:
- Video:Use H264 AVC. Encode the video to a resolution no larger than the screen resolution of the target device (if known).
- Audio: AAC Audio is recommended for all audio resources. AAC achieves better compression at a given quality, compared to mp3 or Ogg Vorbis. Raw formats such as WAV should never be used. The common rational for using the WAV format is that decoding compressed audio streams usually means high latency at playback. However, Android provides the Sound Pool API which enables applications to use compressed audio streams without the penalty of high latency.
- Images: PNG or JPEG. Use PNGs; since it is a lossless format it is very suitable for textures and artwork as there will be no visual artefacts from the compression. If there are space constraints, use JPEGs or a combination of PNGs and JPEGs. A high quality JPEG image may work fine for large photo-realistic images, which the JPEG compression scheme is optimised for.
- Optimise PNG sizes without losing quality
If you use PNG format, PNG images can be reduced in file size without losing quality. To do this, use a tool such as OptiPNG or PNGCrush. Both are great for reducing PNG file size while still ensuring image quality. PNGcrush is an open-source program that iterates over PNG filters and zlib (Deflate) parameters, compresses the image repeatedly using each parameter configuration, and chooses the configuration that yields the smallest compressed (IDAT) output. On the other hand, OptiPNG performs the trials entirely in memory, and writes only the final output file on the disk. Moreover, it offers multiple optimization presets to the user.
5) Remove unused resources Another potential group of space wasters to consider for removal from your APK file are unused resources in your res directory, such as unused layouts, drawables and colors. To detect unused resources in your APK which might be able to be removed, use the android-unused-resources tool. Android Unused Resources is a Java application that will scan your project for unused resources.
6) Avoid duplication Making sure that your application doesn’t have duplicate functionality or duplicate assets is an obvious way to avoid having unnecessary files in your APK. It is important to understand which Android APIs you use, and the full functionality that each provides. It could be that one Android API is already doing the work of another API. Duplicated assets (strings, bitmaps etc) are also a waste of space, and can be easily avoided. To a lesser extent, duplicated code will also unnecessarily increase the size of the delivered binary.
7) Reduce your method count Avid Android developers have longed faced a headache-inducing problem with their apps. The number of methods an app can have is limited. Well, it used to be limited. At long last, the good folks at Google provided solution last October, but it was far from ideal. Though very easy to implement, the multidex solution complicated and prolonged compilation time significantly. Not standing still, starting with Lollipop (Android 5.0), the entire Android Virtual Machine was revolutionized to offer a much more endurable solution, the kind that even improves on the regular compilation time. However, for the time being, the Lollipop solution isn’t ideal too. It requires developers to develop against Lollipop only, which could be problematic since most apps want to be compatible with wider ranges of audiences and might fear using features that aren’t available in earlier Android versions. So right now, the Lollipop solution is a good future-Android solution, but might not have much impact in the foreseeable present. So what can be done in the meantime? We all agree that you shouldn’t give up on features and capabilities, just to avoid reaching the 65K limit. But there is no doubt that the best practice would still be to reduce your application method count. So how can you still try to reduce your method count without losing that little spark that makes your app special?
A Short Overview: “Where are all these methods coming from?”
The method count limit stands roughly at 65K methods (65,536 to be exact). That sounds like a huge number. If you’re a novice Android developer you might be thinking “how on earth can so many apps reach this number so quickly?” You’d probably be somewhat correct. It is not easy to write so many methods on your own. But app developers don’t write their entire app on their own. App developers are using more and more third-party libraries (SDKs – Software Development Kits), which help them achieve certain goals and features that otherwise they’d have to develop completely on their own. From ads through GUI enhancements to social networks, crash reporting and many more, SDKs offer a wide range of capabilities and APIs that save precious development time and help get your app out there faster. But each SDK adds to your method count, and some SDKs are even doing more than just what you bargained for. It’s not necessarily a bad thing, of course. However, the coup de grâce came from Google themselves. Their Google Play Services kit is a large SDK containing over 28,000 methods. With the limit being 65K, that number isn’t exactly low. And many app developers would like to use this SDK since it opens the door to the magical Google kingdom, a much desired capability that adds plenty of value to one’s app. In this post, I won’t only explain how to selectively use the Google Play Services packages, but will also give you the lowdown on how many methods each one of these packages adds to your app. So hold on to your seats, it’s going to be a bumpy ride.
The Google Play Services Playground
So how many methods does Google Play Services actually add? Well, as with everything in life, it’s much more complicated than just a bottom-line number. But let’s take it one step at a time. The Google Play Services SDK actually contains 19 different SDKs. With Gradle, you can selectively choose which ones to use. All you have to do is add the following dependency to your app module’s build.gradle file:
dependencies { compile 'com.google.android.gms:play-services:7.5.0' }
With 7.5.0 being the latest GPS version released at this time. It is labeled as revision 25 inside the Android SDK Manager. In order to choose specific packages, replace the following line with as many dependency lines as you’d like, specifying specific packages. Say for instance, you just want Google Plus, Google Games,Google Ads and Google Maps:
dependencies { compile 'com.google.android.gms:play-services-plus:7.5.0' compile 'com.google.android.gms:play-services-games:7.5.0' compile 'com.google.android.gms:play-services-ads:7.5.0' compile 'com.google.android.gms:play-services-maps:7.5.0' }
Not too difficult, but here’s the first catch. One of the glorious 19 SDKs is called play-services-base. If you include any Google Play Service package, it will automatically be added as well. How many methods does the base package contain? Nearly 8,000 (exact number below).
And here’s the second catch. I didn’t include just 3 packages in the example above. I didn’t even just include 4, if you count the base package as well. In fact, I included 5 packages. Why? Because the Google Games package uses the Google Drive package, and so that was imported as well (in reality,Google Ads also includes a package called tag manager, but I’m only counting the “selectable” packages here).
So now we’re beginning to understand why it’s a little harder to count how many methods each package of the Google Play Services adds to our app. Including one package, does not necessarily mean excluding the others.
So here’s the low-down – which packages you can add, how many methods they add and which other packages do they bring to crash the party. The data refers to the latest version of Google Play Services (7.5.0 / revision 25):
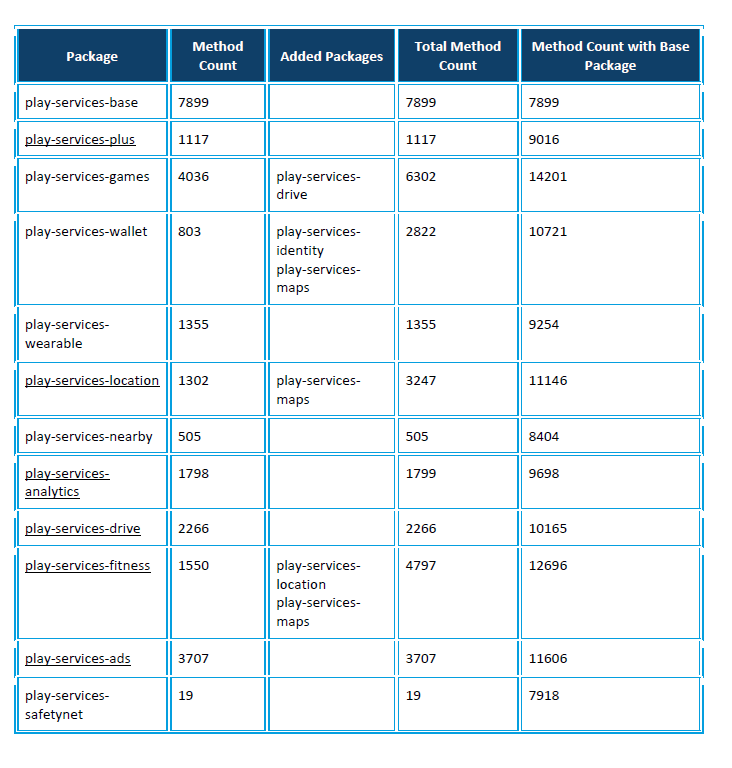
Total number of methods: 28,175.Some of these numbers, you have to admit, are truly atrocious. If all you wanted was the panorama package, you can’t just get nine methods, you have to get almost 8,000. No wonder so many apps are getting close or exceeding that 65K limit.
Some of these numbers may seem to be a bit inconsistent if you try to add them up. That’s because, again, the logic behind Google Play Services is a bit trickier than meets the eye. For instance, Google Analytics includes a single method from Google Ads in order to get the account’s Advertising ID.
Note that when computing how much each package adds to your app, be sure to exclude doubles (for instance, don’t count the base package more than once).
You Can’t Selectively Add Everything
And here’s the final sting. There are two tiny packages inside Google Play Services that cannot be selectively added. The search package (giving you access to the Google Now API) includes only 22 methods. If you want to use it, you don’t have a choice but to add the entire 28,000+ methods, the entire Google Play Services. Hopefully, this will be fixed in the later versions.
(Another “inaccessible” package is the appstate package that includes 111 methods, however it is now deprecated in favor of SavedGames, included in google-play-games package).
I am a “Technical Entrepreneur” having more than 5+ years of experience in the design and development of mobile, web, wearables and desktop apps. I have also worked with Microsoft and Google. I love teaching and have delivered many App development workshops across India.